
C++
Input
Today, we're diving into the world of C++, a widely-used programming language known for its power and efficiency. C++ is an extension of the C programming language, offering object-oriented features and other enhancements. This aiMOOC will guide you through the essentials of C++, from basic syntax to advanced concepts.
Introduction to C++
C++ is a high-level programming language that was developed by Bjarne Stroustrup at Bell Labs in the early 1980s. It's widely used for system/software development, game development, and in embedded systems. The language provides a rich set of features including object-oriented programming, templates, and exception handling.
Basic Syntax and Structure
In C++, a program is made up of functions and variables. The 'main' function is the entry point of every C++ program. Basic syntax includes the use of semicolons (;) to terminate statements and curly braces ({}) to define the scope of functions and control structures.
- C++ Data Types: Integers, floats, characters, and more.
- C++ Operators: Arithmetic, relational, and logical operators.
- Control Structures in C++: If, while, for loops, and switch cases.
Object-Oriented Programming (OOP)
C++ is known for its support of object-oriented programming, which includes concepts like classes, objects, inheritance, polymorphism, and encapsulation.
- Classes and Objects in C++: Understanding the blueprint and instances.
- Inheritance in C++: Base and derived classes.
- Polymorphism in C++: Compile-time and run-time polymorphism.
Advanced Topics
C++ also includes advanced topics that are essential for high-performance and efficient applications.
- Templates in C++: Generic programming with template classes and functions.
- Exception Handling in C++: Try, catch, and throw statements.
- STL in C++: Standard Template Library components like vectors, maps, and algorithms.
Interactive Tasks
Quiz: Test Your Knowledge
What is the entry point of a C++ program? (main function) (!header files) (!class declaration) (!function prototypes)
Which feature is not a part of object-oriented programming in C++? (!Inheritance) (!Polymorphism) (!Templates) (Encapsulation)
Which operator is used for namespace resolution in C++? (::) (!->) (!.) (!#)
What does STL stand for in C++? (Standard Template Library) (!Simple Type Language) (!Standard Type Library) (!Software Tool Library)
Which of these is not a data type in C++? (!int) (!float) (!char) (string)
What keyword is used to define a class in C++? (class) (!struct) (!template) (!function)
Which concept allows multiple functions with the same name but different implementations? (Polymorphism) (!Inheritance) (!Encapsulation) (!Iteration)
What is the primary purpose of a constructor in a class? (To initialize objects of the class) (!To declare variables) (!To return values) (!To end the program)
How are comments marked in C++? (// for single-line, /* / for multi-line) (!#) (!-- --) (!* **)
Which header file is necessary for using I/O operations like cout and cin? (<iostream>) (!<string>) (!<stdlib.h>) (!<math.h>)
Memory
Class | Blueprint for objects |
Object | Instance of a class |
Inheritance | Deriving new classes from existing ones |
Polymorphism | Same function name, different implementations |
Encapsulation | Bundling data and methods that operate on the data |
…
Crossword Puzzle
Inheritance | The concept of basing a new class on an existing class |
Polymorphism | Ability to process objects differently based on their data type or class |
Encapsulation | Hiding internal state and requiring all interaction to be performed through an object's methods |
Namespace | A declarative region that provides a scope to the identifiers inside it |
Template | A feature of C++ that allows functions and classes to operate with generic types |
Algorithm | A step-by-step procedure for calculations, data processing, and automated reasoning |
Vector | A dynamic array in the Standard Template Library |
Exception | An event, which occurs during the execution of a program, that disrupts the normal flow of the program's instructions |
LearningApps
Fill-in-the-Blank
Open Tasks
Easy
- Create a Basic C++ Program: Write a simple C++ program that prints "Hello, World!".
- Explore Data Types: Create variables of different data types and display their values.
Standard
- Implement a Class: Design a simple class with methods and attributes.
- Use Control Structures: Write a program using if-else statements and loops.
Difficult
- Design a Small Project: Build a mini-project like a calculator or a simple game.
- Explore STL: Use the Standard Template Library to solve a complex problem.
Oral Exam
- Discuss OOP Principles: Explain how C++ implements object-oriented programming principles.
- Compare C and C++: Discuss the differences and similarities between C and C++.
- Real-World Applications: Give examples of real-world applications of C++ and explain why it's suitable for these applications.
- Talk about Templates: Discuss the importance of templates in C++ and how they support generic programming.
- STL Importance: Explain why the Standard Template Library is important in C++ programming.
OERs on the Topic
Links
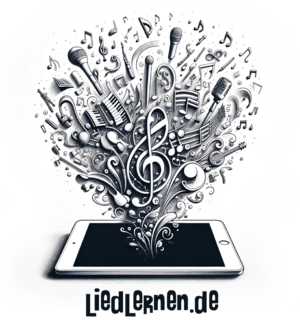
MUSIC QUIZ
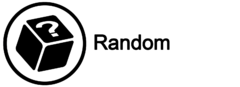









|